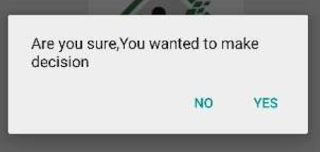
Alert Dialog in android with code examples
Alert dialog in android with code and examples
In this article, we are going to learn about alert dialog in android with code.
In this article we will cover the following topics related to alert dialog in android.
What is Alert Dialog in android?
A dialog is an alert message given to the users while performing an event in small pop up window to perform additional information.
In android you can create the alert dialog by simply creating an instance of Builder.class. Builder class is the inner class of "AlertDialog". You can create instance of Builder class as :
" AlertDialog.Builder instance_of_builder = new AlertDialog.Builder(MainActivity.this);"
here your can pass context as parameter.
After creating instance of alertdialog.builder class you need to set content of the dialog and the action buttons as:
"instance_of_builder.setTitle("This is an Alertdialog from HintAbout");
instance_of_builder.setTitle("Message from HintAbout");
"
Now set the action button like this:
"
instance_of_builder.setPositiveButton("Agree", new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialogInterface, int i) {
Toast.makeText(MainActivity.this,"Agree clicked",Toast.LENGTH_LONG).show();
}
});
instance_of_builder.setNegativeButton("Disagree", (DialogInterface.OnClickListener) null);
"
On more final step is to display the alertdialog:
"
builder.show();
"
Demo of the project code is :
complete code is available at the bottom in github project.
Simple AlertDialog
Following code to make a simple AlertDialog with a title, message and two action button
"
AlertDialog.Builder builder = new AlertDialog.Builder(this); builder.setTitle("Use Google's location services ?"); builder.setMessage("Before proceding to further using google service please agree the policies"); builder.setPositiveButton("Agree", new DialogInterface.OnClickListener() { public void onClick(DialogInterface dialogInterface, int i) { Toast.makeText(MainActivity.this,"Agree clicked",Toast.LENGTH_LONG).show(); } }); builder.setNegativeButton("Disagree", (DialogInterface.OnClickListener) null); builder.show();
"
AlertDialog with Single Choice
Following code is used to make a single choice dialog
String[] strArr = RINGTONE; this.single_choice_selected1 = strArr[0]; AlertDialog.Builder builder = new AlertDialog.Builder(this); builder.setTitle("Phone Ringtone"); builder.setSingleChoiceItems(strArr, 0, new DialogInterface.OnClickListener() { public void onClick(DialogInterface dialogInterface, int i) { String unused = single_choice_selected1 = RINGTONE[i]; } }); builder.setPositiveButton("ok", new DialogInterface.OnClickListener() { public void onClick(DialogInterface dialogInterface, int i) { Toast.makeText(MainActivity.this,"Selected: "+single_choice_selected1,Toast.LENGTH_LONG).show(); } }); builder.setNegativeButton("Cancel", (DialogInterface.OnClickListener) null); builder.show();
AlertDialog with MultiChoice
Following code is used to make a Multichoice dialog
this.single_choice_selected1 = RINGTONE[0]; String[] strArr = COLORS; this.clicked_colors = new boolean[strArr.length]; AlertDialog.Builder builder = new AlertDialog.Builder(this); builder.setTitle("Your preferred colors"); builder.setMultiChoiceItems(strArr, this.clicked_colors, new DialogInterface.OnMultiChoiceClickListener() { public void onClick(DialogInterface dialogInterface, int i, boolean z) { clicked_colors[i] = z; } }); builder.setPositiveButton("Ok", new DialogInterface.OnClickListener() { public void onClick(DialogInterface dialogInterface, int i) { Toast.makeText(MainActivity.this,"Your choice is submited sucessfully",Toast.LENGTH_LONG).show(); } }); builder.setNegativeButton("cancel", (DialogInterface.OnClickListener) null); builder.show();
Copy the following code in your android studio project to clear all doubts about the alert dialog in android.
<linearlayout android:background="@android:color/white" android:fitssystemwindows="true" android:layout_height="fill_parent" android:layout_width="fill_parent" android:orientation="vertical" xmlns:android="http://schemas.android.com/apk/res/android">
<textview android:gravity="center" android:id="@+id/tv_text" android:layout_gravity="center" android:layout_height="wrap_content" android:layout_margintop="40dp" android:layout_width="match_parent" android:text="Example of simple Dialog in android" android:textcolor="@color/colorPrimary" android:textsize="20dp">
<androidx .core.widget.nestedscrollview="" android:id="@+id/nested_scroll_view" android:layout_gravity="center" android:layout_height="wrap_content" android:layout_margintop="50dp" android:layout_width="wrap_content" android:padding="40dp" android:scrollbars="none" android:scrollingcache="true">
<linearlayout android:descendantfocusability="blocksDescendants" android:layout_height="wrap_content" android:layout_width="fill_parent" android:orientation="vertical">
<button android:background="?selectableItemBackground" android:gravity="center|left" android:id="@+id/confirm_dialog" android:layout_height="wrap_content" android:layout_width="fill_parent" android:minheight="?actionBarSize" android:onclick="clickAction" android:paddingleft="@dimen/spacing_large" android:paddingright="@dimen/spacing_large" android:text="CONFIRMATION DIALOG" android:textcolor="@color/grey_60">
<view android:background="@android:color/darker_gray" android:layout_height="1.0dip" android:layout_width="fill_parent"/>
</button >
<button android:background="?selectableItemBackground" android:gravity="center|left" android:id="@+id/alert_dialog" android:layout_height="wrap_content" android:layout_width="fill_parent" android:minheight="?actionBarSize" android:onclick="clickAction" android:paddingleft="@dimen/spacing_large" android:paddingright="@dimen/spacing_large" android:text="ALERT DIALOG" android:textcolor="@color/grey_60"/ >
<button android:background="?selectableItemBackground" android:gravity="center|left" android:id="@+id/single_choice_dialog" android:layout_height="wrap_content" android:layout_width="fill_parent" android:minheight="?actionBarSize" android:onclick="clickAction" android:paddingleft="@dimen/spacing_large" android:paddingright="@dimen/spacing_large" android:text="SINGLE CHOICE DIALOG" android:textcolor="@color/grey_60"/>
<button android:background="?selectableItemBackground" android:gravity="center|left" android:id="@+id/multi_choice_dialog" android:layout_height="wrap_content" android:layout_width="fill_parent" android:minheight="?actionBarSize" android:onclick="clickAction" android:paddingleft="@dimen/spacing_large" android:paddingright="@dimen/spacing_large" android:text="MULTIPLE CHOICE DIALOG" android:textcolor="@color/grey_60"/>
<imageview android:layout_height="200dp" android:layout_width="match_parent" android:src="@drawable/hintaboutlogo"/>
</linearlayout<>/linearlayout >
Complete java program to display alert dialog in android studio
package com.hintAbout.dialogexamples; import android.content.DialogInterface; import android.os.Bundle; import android.view.Menu; import android.view.MenuItem; import android.view.View; import android.widget.Toast; import androidx.appcompat.app.AlertDialog; import androidx.appcompat.app.AppCompatActivity; import androidx.appcompat.widget.Toolbar; public class MainActivity extends AppCompatActivity { private static final String[] COLORS = {"Red", "Green", "Blue", "Purple", "Olive"}; /* access modifiers changed from: private */ public static final String[] RINGTONE = {"None", "Callisto", "Ganymede", "Luna"}; /* access modifiers changed from: private */ public boolean[] clicked_colors = new boolean[COLORS.length]; /* access modifiers changed from: private */ public View parent_view; /* access modifiers changed from: private */ public String single_choice_selected1; /* access modifiers changed from: protected */ public void onCreate(Bundle bundle) { super.onCreate(bundle); setContentView(R.layout.activity_main); this.parent_view = findViewById(R.id.nested_scroll_view); } private void viewsetter() { findViewById(R.id.confirm_dialog).setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { clickAction(view); } }); findViewById(R.id.alert_dialog).setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { clickAction(view); } }); findViewById(R.id.single_choice_dialog).setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { clickAction(view); } }); findViewById(R.id.multi_choice_dialog).setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { clickAction(view); } }); } public void clickAction(View view) { switch (view.getId()) { case R.id.alert_dialog: showAlertDialog(); return; case R.id.confirm_dialog: showConfirmDialog(); return; case R.id.multi_choice_dialog: showMultiChoiceDialog(); return; case R.id.single_choice_dialog: showSingleChoiceDialog(); return; default: return; } } private void showConfirmDialog() { AlertDialog.Builder builder = new AlertDialog.Builder(this); builder.setTitle("Use Google's location services ?"); builder.setMessage("Before proceding to further using google service please agree the policies"); builder.setPositiveButton("Agree", new DialogInterface.OnClickListener() { public void onClick(DialogInterface dialogInterface, int i) { Toast.makeText(MainActivity.this,"Agree clicked",Toast.LENGTH_LONG).show(); } }); builder.setNegativeButton("Disagree", (DialogInterface.OnClickListener) null); builder.show(); } private void showAlertDialog() { AlertDialog.Builder builder = new AlertDialog.Builder(this); builder.setTitle("Discard draft ?"); builder.setPositiveButton("Discard", new DialogInterface.OnClickListener() { public void onClick(DialogInterface dialogInterface, int i) { Toast.makeText(MainActivity.this,"Discard clicked",Toast.LENGTH_LONG).show(); } }); builder.setNegativeButton("Cancel", (DialogInterface.OnClickListener) null); builder.show(); } private void showSingleChoiceDialog() { String[] strArr = RINGTONE; this.single_choice_selected1 = strArr[0]; AlertDialog.Builder builder = new AlertDialog.Builder(this); builder.setTitle("Phone Ringtone"); builder.setSingleChoiceItems(strArr, 0, new DialogInterface.OnClickListener() { public void onClick(DialogInterface dialogInterface, int i) { String unused = single_choice_selected1 = RINGTONE[i]; } }); builder.setPositiveButton("ok", new DialogInterface.OnClickListener() { public void onClick(DialogInterface dialogInterface, int i) { Toast.makeText(MainActivity.this,"Selected: "+single_choice_selected1,Toast.LENGTH_LONG).show(); } }); builder.setNegativeButton("Cancel", (DialogInterface.OnClickListener) null); builder.show(); } private void showMultiChoiceDialog() { this.single_choice_selected1 = RINGTONE[0]; String[] strArr = COLORS; this.clicked_colors = new boolean[strArr.length]; AlertDialog.Builder builder = new AlertDialog.Builder(this); builder.setTitle("Your preferred colors"); builder.setMultiChoiceItems(strArr, this.clicked_colors, new DialogInterface.OnMultiChoiceClickListener() { public void onClick(DialogInterface dialogInterface, int i, boolean z) { clicked_colors[i] = z; } }); builder.setPositiveButton("Ok", new DialogInterface.OnClickListener() { public void onClick(DialogInterface dialogInterface, int i) { Toast.makeText(MainActivity.this,"Your choice is submited sucessfully",Toast.LENGTH_LONG).show(); } }); builder.setNegativeButton("cancel", (DialogInterface.OnClickListener) null); builder.show(); } }
Manifest file code for the project
Gradle code for the project
<manifest package="com.hintabout.dialogexamples" xmlns:android="http://schemas.android.com/apk/res/android">
<application android:allowbackup="true" android:icon="@mipmap/ic_launcher" android:label="@string/app_name" android:roundicon="@mipmap/ic_launcher_round" android:supportsrtl="true" android:theme="@style/AppTheme">
<activity android:name=".MainActivity">
<intent-filter>h;
<action android:name="android.intent.action.MAIN">
<category android:name="android.intent.category.LAUNCHER">
</category>h; </action > </intent-filter >
</activity >
</application>
</manifest >
apply plugin: 'com.android.application' android { compileSdkVersion 28 defaultConfig { applicationId "com.hintbabout.dialogexamples" minSdkVersion 18 targetSdkVersion 28 versionCode 1 versionName "1.0" testInstrumentationRunner "androidx.test.runner.AndroidJUnitRunner" } buildTypes { release { minifyEnabled false proguardFiles getDefaultProguardFile('proguard-android-optimize.txt'), 'proguard-rules.pro' } } } dependencies { implementation fileTree(dir: 'libs', include: ['*.jar']) implementation 'androidx.appcompat:appcompat:1.3.0' implementation 'androidx.constraintlayout:constraintlayout:2.0.4' testImplementation 'junit:junit:4.12' androidTestImplementation 'androidx.test.ext:junit:1.1.3' androidTestImplementation 'androidx.test.espresso:espresso-core:3.4.0' }
For Complete code check it on our GitHub account: https://github.com/GhayasAhmed786/AndroidBasicDialog.git
Thanks for reading our article.
Thanks for reading our article.
0 Response to "Alert Dialog in android with code examples"
Post a Comment